We’ll explore the ins and outs of Canvas in this article, demonstrating what is possible with examples and links.
Why Do You Need Canvas?
Canvas can be used to represent something visually in your browser. For example:
» Simple diagrams
» Fancy user interfaces
» Animations
» Charts and graphs
» Embedded drawing applications
» Working around CSS limitations
In basic terms it’s a very simple pixel-based drawing API, but used in the right way it can be the building block for some clever stuff.
What Tools Are at Your disposal?
Drawing tools
» Rectangles
» Arcs
» Paths and line drawing
» Bezier and quadratic curves
» Effects
Fills and strokes
» Shadows
» Linear and radial gradients
» Alpha transparency
» Compositing
» Transformations
» Scaling
» Rotation
» Translation
» Transformation matrix
Getting data in and out
» Loading external images by URL, other canvases or data URI
» Retrieving a PNG representation of the current canvas as a data URI
The excellent Canvas cheat sheet is a great reference of the commands available.
Getting Started
To use Canvas, you’ll need two things
» A Canvas tag in the HTML to place the drawing canvas
» JavaScript to do the drawing
The Canvas tag is basically an img tag without any data. You specify a width and a height for the drawing area. Instead of an alt attribute, you can enclose HTML within the canvas tag itself for alternative content.
Example of a Canvas tag:
<canvas id="myDrawing" width="200" height="200">
<p>Your browser doesn't support canvas.</p>
</canvas>
With a Canvas element in the HTML, we’ll add the JavaScript. We need to reference the Canvas element, check the browser is Canvas-compatible and create a drawing context:
var drawingCanvas = document.getElementById('myDrawing');
// Check the element is in the DOM and the browser supports canvas
if(drawingCanvas.getContext) {
// Initaliase a 2-dimensional drawing context
var context = drawingCanvas.getContext('2d');
//Canvas commands go here
}
Checking for the getContext() method on a canvas DOM object is the standard way of determining canvas compatibility.
The context variable now references a Canvas context and can be drawn on.
Basic Drawing
So, let’s get started with an example to demonstrate the basics. We’re going to draw a smiley face, similar to the one on the Acid2 reference rendering.
If we think about the face as a set of basic shapes, we have:
1. A circle, with a black stroke and yellow fill for the face.
2 circles with a black stroke and white fill and with an inner circle of filled green for the eyes.
3. A curve for the smile.
4. A diamond for the nose.
Let’s start by creating the round face:
// Create the yellow face
context.strokeStyle = "#000000";
context.fillStyle = "#FFFF00";
context.beginPath();
context.arc(100,100,50,0,Math.PI*2,true);
context.closePath();
context.stroke();
context.fill();
You can see from the above that we start by defining some colours for the stroke and fill, then we create a circle (an arc with a radius of 50 and rotated through the angles of 0 to 2*Pi radians). Finally, we apply the stroke and fill that has been defined previously.
This process of setting styles, drawing to co-ordinates and dimensions and finally applying a fill or stroke is at the heart of Canvas drawing. When we add the other face elements in, we get:

Download the full source code of the smiley face example
Effects and Transformations
Let’s see how we can manipulate an existing image in canvas. We can load external images using the drawImage() method:
context.drawImage(imgObj, XPos, YPos, Width, Height);
Here’s the image we’re going to play with:

We’re going to give the image a red frame. For this we’ll use a rectangle and fill it with a radial gradient to give it a bit of texture.
//Create a radial gradient.
var gradient = context.createRadialGradient(90,63,30,90,63,90);
gradient.addColorStop(0, '#FF0000');
gradient.addColorStop(1, '#660000');
//Create radial gradient box for picture frame;
context.fillStyle = gradient;
context.fillRect(15,0,160,140);
//Load the image object in JS, then apply to canvas onload
var myImage = new Image();
myImage.onload = function() {
context.drawImage(myImage, 30, 15, 130, 110);
}
myImage.src = "cocktail.jpg";
To make our portrait look like it’s hung on a wall, we’ll add a drop shadow and rotate the image slightly so it looks at a slight angle.
A drop shadow will need to be applied to the frame.
//Create a drop shadow
context.shadowOffsetX = 10;
context.shadowOffsetY = 10;
context.shadowBlur = 10;
context.shadowColor = "black";

Download the full source code of the portrait example
To rotate the canvas, we use the rotate() method which takes a value in radians to rotate the canvas by. This only applies rotation to subsequent drawing to the canvas, so make sure you’ve put this in the right place in your code.
//Rotate picture
context.rotate(0.05);
This is what the finished frame looks like:
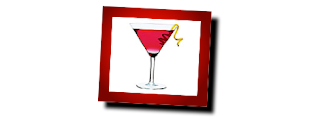
Download the full source code of the portrait example
Source : http://thinkvitamin.com/dev/html-5-dev/how-to-draw-with-html-5-canvas/
For Website Designing and Development
Web designer Hyderabad
eMail : varadesigns@yahoo.com
phone : +91 9676739333